SOAPing Up Web Services
We discuss the WSDL specification and how it works with SOAP, and show key samples of code.
October 14, 2002
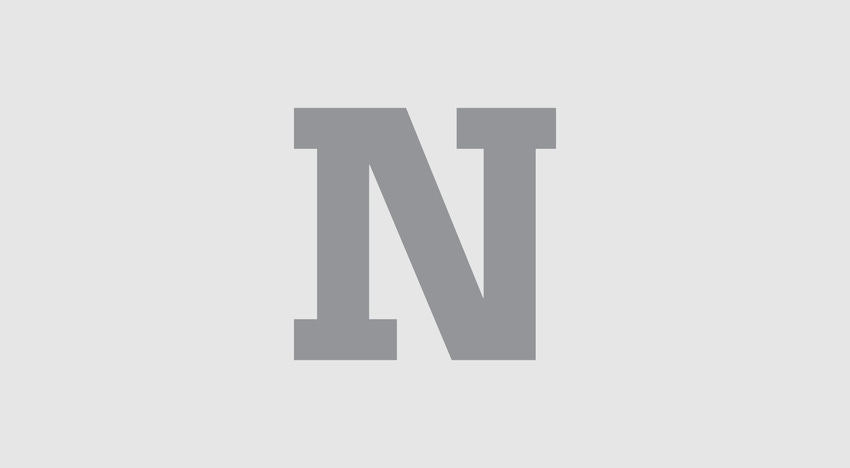
The How
Deploying Web Services can be tricky. Contrary to the hype from your favorite vendor, this is a new, somewhat bumpy way of doing things. The general steps are: write a Web service in your favorite development environment; generate the Web services wrapper from the source code (Microsoft .Net will do this step for you if you let it); generate a server deployment package and the server's WSDL (Web Services Definition Language) definition from the Web services wrapper; generate a client "stub" package from the server's WSDL file; and deploy and test your Web service.
Of course, to test it you'll have to write a client, so the next step is to write a client using the client "stub" file to generate the interface class. Sounds like a lot of work, huh? Luckily most of the process is automated when you use Apache/Tomcat and Microsoft IIS/.Net.
Step By Step: How a Web Service is Called 1. A client application requests a URI (universal resource identifier) using a SOAP-encoded message. |
The WSDL specification offers a system-independent way for your application to say, "If you want to talk to me, here's how you have to do it." System independence comes from writing the definition in an XML-formatted file. The SOAP (Simple Object Access Protocol) specification, on the other hand, lets your application communicate with client applications. WSDL says, "When you call me, you should have X, Y and Z." SOAP is how X, Y and Z get to the application, and how it returns its response.
Most SOAP exchanges take place over HTTP, and most Web services define HTTP as their "transport mechanism," but this is by no means required. WSDL can set any of several transport mechanisms as the way to call your application and how your applications can respond. For example, you may have a Web services client that says, "Close out the books for the year," and sends the request over HTTP. A year-end close-out is no trivial task, and obviously you're not going to wait with your Web browser for the task to end. Therefore the application may say in WSDL, "If we're closing out the books, the response mechanism will be SMTP; I'll send an e-mail to the boss letting him know when we're done."
Server stubs are the small pieces of code that tell the application server about your application and let the server communicate with your app. Client stubs tell the client application about your interfaces in a system-dependent manner--they are generated from WSDL, which is system-independent, so think of them as the clients' translation of your interfaces into their programming language.
You can create a WSDL file for Web services by using one of many free applications. "Java2wsdl" (from Apache.org) for Java Web services and "WSDL.exe" for .Net Web services are the two most popular generators and will take a written application and generate the WSDL file for it. From this WSDL file you can then generate server and client stubs to use with projects to deploy and access your Web services.
Web services consist of a calling specification, an interface specification, a response specification and the program that does the work.
• The Calling Specification: The minimum sequence of events required to communicate with a Web server (assuming, for simplicity, that HTTP is the transport for both directions) is as follows:
1. The client sends an HTTP post request that includes the Web service and the parameters. Note that an HTTP get specification is also available.
2. The server sends an HTTP response that includes the result of the processing requested.
• The Interface Specification: WSDL: You already know that WSDL tells the world how to contact your application, but you can also think of WSDL as a standard--in the sense that a phone number is a standard. You must know the phone number to communicate with the person you're calling. Likewise, your application must know the interface to communicate with a Web service. Most environments will generate WSDL files automatically--you shouldn't have to write them.
So how do you get your hands on the WSDL file of a Web service? Most will respond to a request of the form: www.mywebservice.com/someServiceURL/wsdl?
FYI There are excellent tutorials on generating WSDL and Web service wrappers for both .Net and Java/Tomcat Web services. O'Reilly offers one for Java applications, "Creating Web Services with Apache Axis," at OnJava.com, and Microsoft has an excellent set of samples here. |
Say a vendor has told you about its Wonderful Web Service; just go to the URL provided and add "/wsdl?" The response should be an XML document in the format discussed in this article.
On Your Level
Understanding the layout of a WSDL file is straightforward--if you take it a level at a time. Here, in an abstract format, is how to read WSDL files and determine the interface that a Web service provides.
• Level 1: There are only two major elements at this level: which version of XML is supported and the top-level definition statement.
XML Version: The version of the W3C XML specification to which this document conforms.
Definitions: This is where you define your service and any supporting data types. All of Level 2 fits into this piece of Level 1.
• Level 2: The Service Definition
|
Types: Here you define the complex data types used by the rest of the file. For example, if the output from a Web service defined in this file is a date, a time and a value, such as stock price, you will need to define the tags and XML data types for these output fields.
Message Name: In this section you define the messages your program knows how to process. It is a mapping from the external name to the internal name.
Port Type: This is the public entry point for calling applications. It defines what operations are available and what messages are used by the operations.
Binding Name: This section binds an operation to a transport mechanism. This is where you say "requests come via HTTP post messages to this URL, and responses come back via SMTP messages."
Service Name: This is the public advertisement for your service. It tells the service name and where to find it, and possibly documents it in readable form.
Level 3 further defines Level 2 elements. This is where you would say, "The transport mechanism for this Level 2 Binding Name is HTTP." Level 3 is different for each Level 2 element, so we will not explore it here.
In summary, WSDL offers three important pieces of information to the client: What messages it recognizes, what transport mechanism it uses to communicate those messages and how to contact this service. The following example is simplified from the SOAP committee's WSDL 1.1 Specification Document:
Recommended Reading • WSDL (Web Services Definition Language) |
First, at what URL are these services located? This is in the "soap:Address location" field of the service definition of the WSDL file:
What messages does this Web service understand? To find out, we examine the definition for a message called "GetLastTradePriceInput," which takes a ticker symbol as input. Note that "Message Name" is defined as a Level 2 element. It has (in this example) a single Level 3 element called "part name."
And finally, "What information can I expect to get back, and what format will it be in?"
The response the server will send is called "GetLastTradePriceOutput," and it passes a floating point number:
As we touched upon earlier, SOAP is used to send a message to a Web service and get a response. It employs XML to provide the layer of system independence Web services hope to achieve, defining a way to express URLs and parameters that can be mapped into system-dependent variables by both the caller and the service.
The header for a SOAP message bound to HTTP looks just like any other HTTP post header with a content-type of text/XML. The body of the post message holds the "SOAP Envelope," which contains the request to the service. This consists of an optional header, any name spaces to use, and the actual call with parameters, all in plain-text XML. The response is set up exactly the same way, but the results of the service with your parameters are the contents of the message (see "Example of Client SOAP Message Over HTTP" below).
Note how the (simplified) example is structured. The normal HTTP headers are present, followed by the SOAP Envelope version, the SOAP Encoding Style and the SOAP body--our area of interest. Our Web Service has an entry point defined at www.Someplace.org/GetSomeValues. This entry point takes a single string as input--we sent "test input" as the string with the XML tag paramOne.
The server processes the request and sends the following response shown in "Example of Server Response." Note again the normal HTTP header. For a "200 OK" message, the content length--if present--must be exact according to the SOAP spec. This is in line with HTTP 1.1 standards but could cause problems on some HTTP 1.0 servers. The normal content type header says "text/XML," the SOAP header includes encoding information for both the message and the header, and the SOAP body is where the "payload" is. The routine is "getSomeValueResponse," and the ResponseText is in the "Result" tag.
Web Links • "W3C, Oasis Look for Common Web Services Ground" (InternetWeek Aug. 28, 2002)
|
SOAP also defines the system-independent data types allowed for communications in the Web services world and provides a simple mechanism for building abstract data types out of them.
Putting It All Together
you have a working application and the WSDL file that describes its interface. What else do you need? For starters, you need to deploy the application and publish your WSDL file. Given that you are in an all .Net environment, Microsoft makes this task trivial--assuming you accept all the defaults. While a Tomcat/Apache installation requires more work, at this stage in the Web services standard, we feel more work is good. If you know how the pieces interoperate, you will understand the system better and can more easily debug it.
When the time comes to implement Web services, you need to know how to deploy them and how to build clients that use them. For most, it will be enough to understand what the request and reply packets are and how to read the main portions of WSDL files.
Don MacVittie is an applied technologist at WPS Resources. Write to him at [email protected].
Read more about:
2002